A more advanced way to play animations with FlexMotion is to use its dedicated asset types. These are called FlexMotion (hence the name of the package). They are ScriptableObject based data containers referencing one or multiple animation clips and various settings. Similar to an AnimationClip, they can be played by the FlexMotionAnimator.
FlexMotion assets come in four different varieties:
- Solo: Referencing only one Animation clip.
- 1-dimensional: Referencing an array of Animation clips. Blending is based on their weights, which are computed via a dedicated method from a single float value and the clips order in the array. The weights can also be manually set.
- 2-dimensional 4-Ways: Referencing 5 Animation clips corresponding to 4 cardinal directions and one neutral state. Similar to the 1D type, the weights are computed via a dedicated method but using a Vector2 instead.
- 2-dimensional 8-Ways: Same as the 2D 4 ways variant but with 8 cardinal directions instead of 4.
In summary, these are especially useful when you need to blend multiple animations into one singular motion and / or have pre-defined motion layer settings.
To create a FlexMotionLayer, use the Project window's create asset menu, go to FlexMotion and the select the type you want.
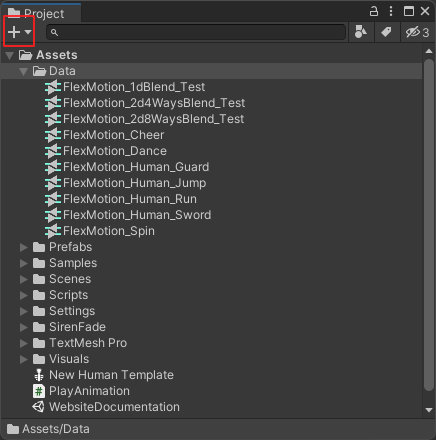
When it comes to using them, it's exactly the same as playing Animation clips. Here is a simple example:
using SV.FlexMotion;
using UnityEngine;
public class PlayFlexMotionAsset : MonoBehaviour
{
[SerializeField]
private FlexMotionAnimator animator;
[SerializeField]
private FlexMotion flexMotion;
private void Start()
{
animator.Play(flexMotion);
}
}
Note that the FlexMotion asset types inherit from the same abstract class, so you don't have to specify the type you are using on the serialized field.
Using these containers is similar to playing an animation clip. Therefore, you can refer to the Play animation clips section of the documentation. The only key difference is that the settings are applied on the motion layer upon calling the Play method. You can still change all these settings using method chaining or any other way if needed.
To learn how to use the blending methods, refer to the documentation pages of the 1D, 2D 4 ways and 2D 8 ways FlexMotion assets.
Child Pages
Flexmotion Solo
The simplest FlexMotion container. It allows you to pre-set some settings that will be applied when played.
Flexmotion 1D
A FlexMotion container that allows animation blending via the modification of the FlexMotionLayer's blend weights.
Flexmotion 2D 4Ways
A FlexMotion container that allows animation blending via the modification of the FlexMotionLayer's blend weights.
Flexmotion 2D 8Ways
All information covered in the FlexMotion 1D and FlexMotion 2D 4 Ways is applicable for this variant.