The simplest way to use FlexMotion is to use the FlexMotionAnimator's Play() method with an animation clip.
Here is a simple component script to do just that:
using SV.FlexMotion;
using UnityEngine;
public class PlayAnimation : MonoBehaviour
{
[SerializeField]
private FlexMotionAnimator animator;
[SerializeField]
private AnimationClip clip;
private void Start()
{
animator.Play(clip);
}
}
After creating that script, add that component to a gameobject, return to the editor and assign the exposed fields. Finally enter play mode and your gameobject should be animated like so:
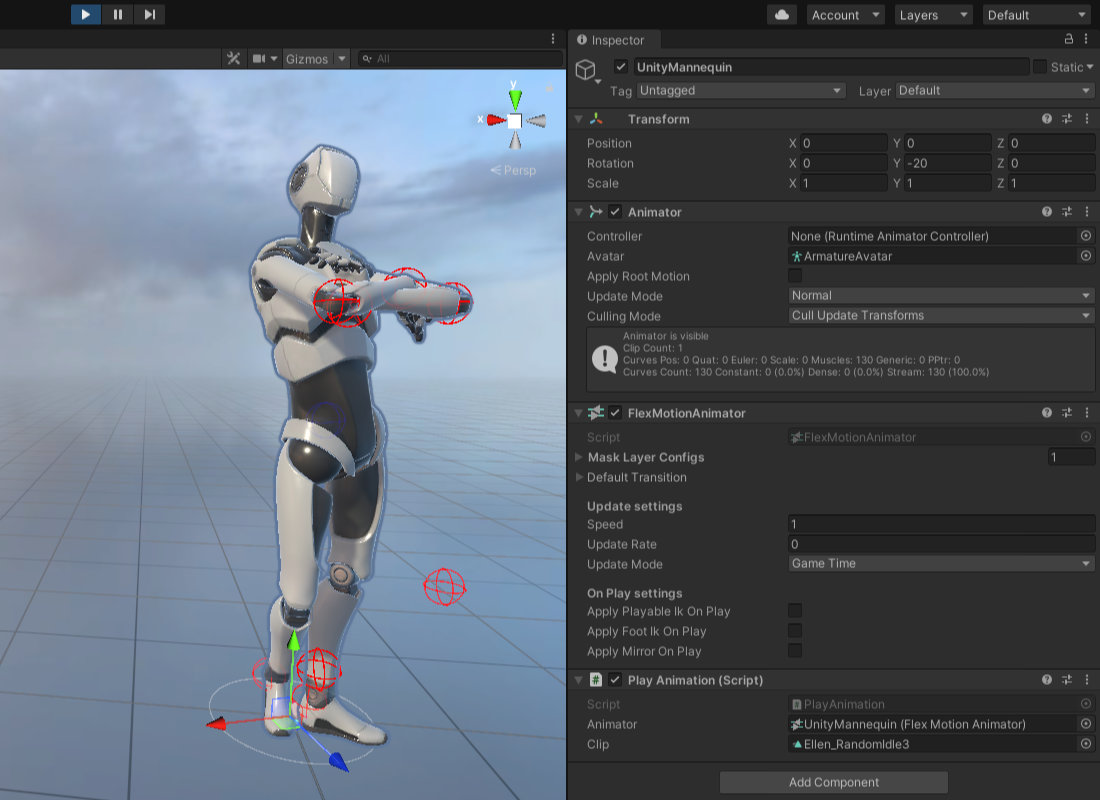
📝 Notice
If no animation is currently playing (which is the case when playing the first animation), the transition will be skipped.
While this is already quite useful, FlexMotion allows you to do way more.
The Play() method returns the Motion Layer instance that will play the animation. Different settings are available on a Motion Layer and can be modified freely.
One way to do that is to call the Motion Layer dedicated setting methods. Leveraging the fact that these methods are returning the called Motion Layer itself, we can use a technique called Method Chaining.
To illustrate that here is a modified version of the previous script that will play the animation clip faster and mirror it:
using SV.FlexMotion;
using UnityEngine;
public class PlayAnimation : MonoBehaviour
{
[SerializeField]
private FlexMotionAnimator animator;
[SerializeField]
private AnimationClip clip;
private void Start()
{
animator.Play(clip).SetSpeed(2.0f).SetIsMirror(true);
}
}
Entering play mode should result in the animation playing twice as fast and being mirrored like so:
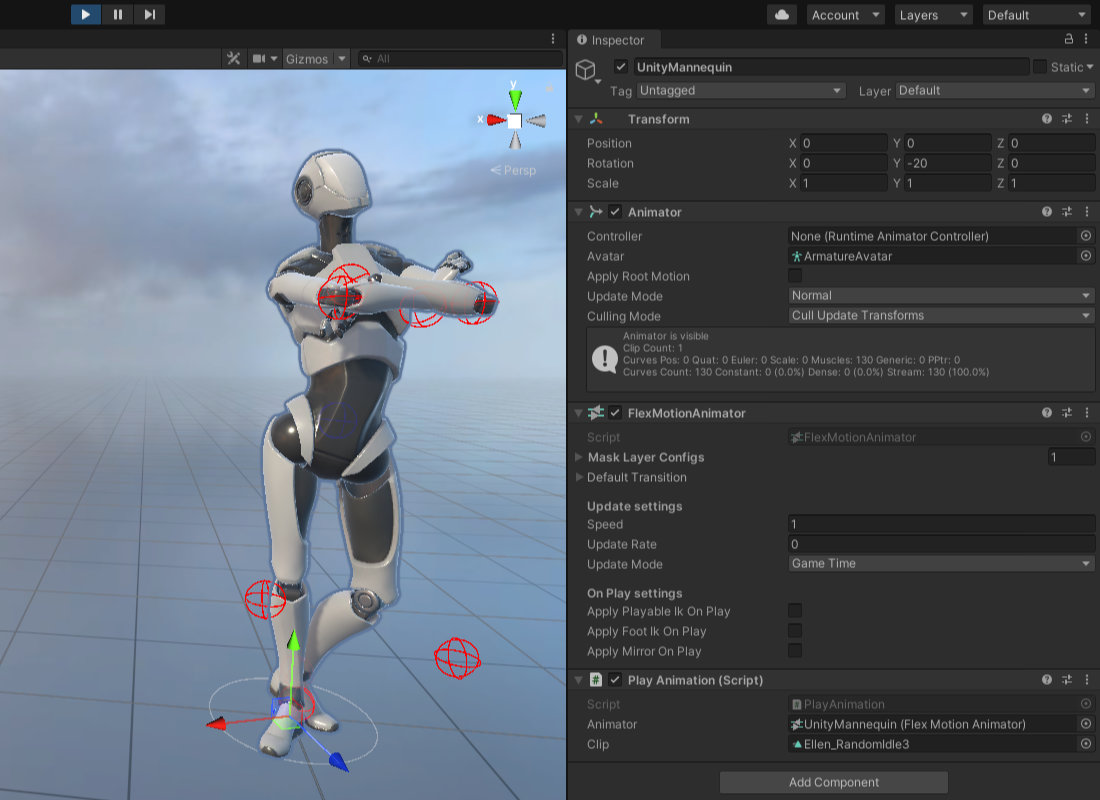
It is also possible to keep the motion layer reference and modifying it later. Here is an example:
using SV.FlexMotion;
using UnityEngine;
public class PlayAnimation : MonoBehaviour
{
[SerializeField]
private FlexMotionAnimator animator;
[SerializeField]
private AnimationClip clip;
private FlexMotionLayer _layer;
private void Start()
{
_layer = animator.Play(clip);
}
[ContextMenu("Mirror")]
public void Mirror()
{
_layer.IsMirror = !_layer.IsMirror;
}
}
Thanks to the ContextMenu attribute, you can now right-click on the PlayAnimation component and select "Mirror" while in play mode.
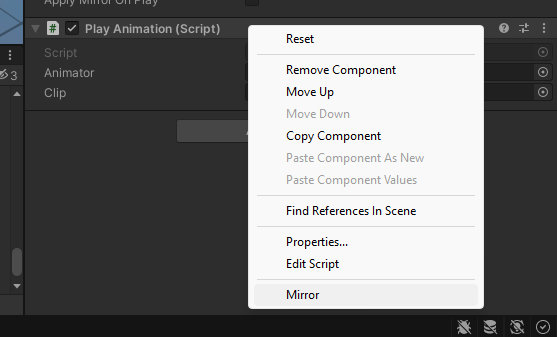
This will mirror the animated object every time you use that menu.
📝 Notice
The Motion Layer states will change if a new animation is being played. The layer will be stopped and eventually reused. To avoid this issue, you can manage the Motion Layer settings just after calling the Play() method or use the OnStop callback to determine its state.